Lombok Builder Inheritance Workaround
Lombok is a Java library that automatically generates code based on annotations. The @Builder annotation can be used to create a builder class for a class with many fields. However, the @Builder annotation does not support inheritance. This means that if a class extends another class that has a builder, the subclass will not be able to use the builder of the superclass. The Lombok Builder Inheritance Workaround is a technique that can be used to work around this limitation. The workaround involves creating a new builder class for the subclass that delegates to the builder of the superclass.
The Lombok Builder Inheritance Workaround has several benefits. First, it allows subclasses to use the builder of the superclass. This can save time and effort, as the subclass does not have to define its own builder class. Second, it ensures that the builder of the subclass is consistent with the builder of the superclass. This can help to prevent errors and maintain a consistent code style.
The Lombok Builder Inheritance Workaround is a simple and effective way to work around the limitations of the @Builder annotation. It can save time and effort, and it can help to ensure that the builder of the subclass is consistent with the builder of the superclass.
Lombok Builder Inheritance Workaround
The Lombok Builder Inheritance Workaround is a technique that allows subclasses to use the builder of the superclass. This can save time and effort, and it can help to ensure that the builder of the subclass is consistent with the builder of the superclass.
- Delegation: The workaround involves creating a new builder class for the subclass that delegates to the builder of the superclass.
- Inheritance: The new builder class inherits from the builder class of the superclass.
- Consistency: The new builder class ensures that the builder of the subclass is consistent with the builder of the superclass.
- Extensibility: The workaround allows subclasses to extend the functionality of the builder of the superclass.
- Reusability: The workaround allows subclasses to reuse the code of the builder of the superclass.
- Simplicity: The workaround is simple and easy to implement.
- Efficiency: The workaround can save time and effort.
- Effectiveness: The workaround can help to ensure that the builder of the subclass is consistent with the builder of the superclass.
The Lombok Builder Inheritance Workaround is a valuable tool for Java developers. It can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Delegation
The delegation mechanism is a fundamental aspect of the Lombok Builder Inheritance Workaround. It allows subclasses to inherit the functionality of the builder of the superclass, while still being able to define their own custom builder behavior. This can save time and effort, and it can help to ensure that the builder of the subclass is consistent with the builder of the superclass.
In order to implement delegation, the new builder class for the subclass must inherit from the builder class of the superclass. This will give the new builder class access to all of the methods and fields of the superclass's builder class. The new builder class can then override any of the methods of the superclass's builder class in order to customize the behavior of the builder. For example, the new builder class could add new methods to the builder, or it could change the way that existing methods work.
The Lombok Builder Inheritance Workaround is a powerful tool that can be used to extend the functionality of the Lombok @Builder annotation. It allows subclasses to reuse the code of the builder of the superclass, while still being able to define their own custom builder behavior. This can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Inheritance
Inheritance is a fundamental concept in object-oriented programming. It allows a new class (subclass) to inherit the properties and methods of an existing class (superclass). In the context of the Lombok Builder Inheritance Workaround, the new builder class inherits from the builder class of the superclass. This means that the new builder class has access to all of the methods and fields of the superclass's builder class.
The Lombok Builder Inheritance Workaround is a technique that allows subclasses to use the builder of the superclass. This can save time and effort, and it can help to ensure that the builder of the subclass is consistent with the builder of the superclass. The workaround involves creating a new builder class for the subclass that delegates to the builder of the superclass. The new builder class inherits from the builder class of the superclass, and it can override any of the methods of the superclass's builder class in order to customize the behavior of the builder.
For example, consider the following code:
javaclass Superclass { private String name; private int age; @Builder public Superclass(String name, int age) { this.name = name; this.age = age; }}class Subclass extends Superclass { private String address; @Builder public Subclass(String name, int age, String address) { super(name, age); this.address = address; }}
In this example, the `Subclass` inherits from the `Superclass`. The `Superclass` has a builder class that is annotated with the `@Builder` annotation. The `Subclass` also has a builder class that is annotated with the `@Builder` annotation. The `Subclass`'s builder class inherits from the `Superclass`'s builder class. This means that the `Subclass`'s builder class has access to all of the methods and fields of the `Superclass`'s builder class. The `Subclass`'s builder class can also override any of the methods of the `Superclass`'s builder class in order to customize the behavior of the builder.
The Lombok Builder Inheritance Workaround is a powerful tool that can be used to extend the functionality of the Lombok `@Builder` annotation. It allows subclasses to reuse the code of the builder of the superclass, while still being able to define their own custom builder behavior. This can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Consistency
The Lombok Builder Inheritance Workaround ensures that the builder of the subclass is consistent with the builder of the superclass. This is important because it helps to maintain a consistent coding style throughout the project. It also helps to prevent errors, as the builder of the subclass will be able to use the same methods and fields as the builder of the superclass.
- Reduced Errors
By ensuring consistency between the builder of the subclass and the builder of the superclass, the Lombok Builder Inheritance Workaround helps to reduce errors. This is because the builder of the subclass will be able to use the same methods and fields as the builder of the superclass. This reduces the chances of making mistakes when using the builder.
- Improved Code Maintainability
The Lombok Builder Inheritance Workaround also helps to improve code maintainability. This is because it ensures that the code is consistent throughout the project. This makes it easier to understand and maintain the code.
- Increased Reusability
The Lombok Builder Inheritance Workaround can also increase the reusability of code. This is because the builder of the subclass can be reused by other subclasses. This can save time and effort when developing new code.
- Enhanced Extensibility
The Lombok Builder Inheritance Workaround can also enhance the extensibility of code. This is because it allows subclasses to extend the functionality of the builder of the superclass. This can be useful when adding new features to the code.
Overall, the Lombok Builder Inheritance Workaround is a valuable tool that can help to improve the quality of your code. It can help to reduce errors, improve code maintainability, increase reusability, and enhance extensibility.
Extensibility
The Lombok Builder Inheritance Workaround allows subclasses to extend the functionality of the builder of the superclass. A subclass can add new methods to the builder or override existing methods to change their behavior. This is useful when the subclass needs to add new features or change the behavior of the builder.
For example, consider the following code:
java class Superclass { private String name; @Builder public Superclass(String name) { this.name = name; } } class Subclass extends Superclass { private int age; @Builder public Subclass(String name, int age) { super(name); this.age = age; } }
In this example, the `Subclass` extends the `Superclass` and adds a new field called `age`. The `Subclass` also overrides the `Builder` method to add a new parameter for the `age` field. This allows the `Subclass` to create builders that can set both the `name` and `age` fields.
The ability to extend the functionality of the builder of the superclass is a powerful feature of the Lombok Builder Inheritance Workaround. It allows subclasses to add new features or change the behavior of the builder without having to modify the superclass.
The Lombok Builder Inheritance Workaround is a valuable tool for Java developers. It can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Reusability
The Lombok Builder Inheritance Workaround allows subclasses to reuse the code of the builder of the superclass. This is a powerful feature that can save time and effort, and it can help to ensure that the code is consistent and maintainable.
One of the main benefits of reusing code is that it can reduce the amount of time it takes to develop new software. This is because developers do not have to write the same code multiple times. Instead, they can simply reuse code that has already been written and tested.
Reusing code can also help to improve the quality of the code. This is because reused code has already been tested and debugged, so it is less likely to contain errors. Additionally, reused code is more likely to be consistent with the rest of the code in the project, which can help to improve the overall quality of the software.
The Lombok Builder Inheritance Workaround makes it easy to reuse the code of the builder of the superclass. This is because the workaround allows subclasses to inherit the builder of the superclass. This means that subclasses can use the same builder to create instances of themselves as the superclass. This can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Here is an example of how the Lombok Builder Inheritance Workaround can be used to reuse the code of the builder of the superclass:
java class Superclass { private String name; @Builder public Superclass(String name) { this.name = name; } } class Subclass extends Superclass { private int age; @Builder public Subclass(String name, int age) { super(name); this.age = age; } }
In this example, the `Subclass` reuses the code of the builder of the `Superclass`. This is because the `Subclass` inherits the builder of the `Superclass`. This allows the `Subclass` to use the same builder to create instances of itself as the `Superclass`. This saves time and effort, and it helps to ensure that the code is consistent and maintainable.
Simplicity
The simplicity of the Lombok Builder Inheritance Workaround is one of its key advantages. The workaround is based on a simple delegation mechanism that is easy to understand and implement. This makes the workaround accessible to developers of all skill levels, and it can be used in a wide variety of projects.
The simplicity of the workaround also makes it easy to maintain. As the superclass's builder class changes, the subclass's builder class will automatically update to reflect those changes. This eliminates the need for developers to manually update the subclass's builder class, which can save time and effort.
The simplicity of the Lombok Builder Inheritance Workaround is a major factor in its popularity. The workaround is a valuable tool that can save developers time and effort, and it can help to improve the quality of the code.
Efficiency
The Lombok Builder Inheritance Workaround can save time and effort in several ways. First, it eliminates the need to write boilerplate code to create a builder class for a subclass. This can save a significant amount of time, especially for subclasses with a large number of fields.
- Reduced Boilerplate Code
By eliminating the need to write boilerplate code, the Lombok Builder Inheritance Workaround can save developers a significant amount of time. This is especially beneficial for subclasses with a large number of fields.
- Improved Code Maintainability
The Lombok Builder Inheritance Workaround can also improve code maintainability. This is because it ensures that the builder class for a subclass is consistent with the builder class for the superclass. This makes it easier to understand and maintain the code.
- Increased Reusability
The Lombok Builder Inheritance Workaround can also increase the reusability of code. This is because it allows subclasses to reuse the builder class of the superclass. This can save time and effort when developing new code.
- Enhanced Extensibility
The Lombok Builder Inheritance Workaround can also enhance the extensibility of code. This is because it allows subclasses to extend the functionality of the builder class of the superclass. This can be useful when adding new features to the code.
Overall, the Lombok Builder Inheritance Workaround is a valuable tool that can save developers time and effort. It can also improve the quality of the code by reducing boilerplate code, improving code maintainability, increasing reusability, and enhancing extensibility.
Effectiveness
The Lombok Builder Inheritance Workaround is an effective way to ensure that the builder of the subclass is consistent with the builder of the superclass. This is important because it helps to maintain a consistent coding style throughout the project. It also helps to prevent errors, as the builder of the subclass will be able to use the same methods and fields as the builder of the superclass.
For example, consider the following code:
javaclass Superclass { private String name; @Builder public Superclass(String name) { this.name = name; }}class Subclass extends Superclass { private int age; @Builder public Subclass(String name, int age) { super(name); this.age = age; }}
In this example, the `Subclass` extends the `Superclass` and adds a new field called `age`. The `Subclass` also overrides the `Builder` method to add a new parameter for the `age` field. This ensures that the builder of the `Subclass` is consistent with the builder of the `Superclass`. This means that the `Subclass` can use the same builder to create instances of itself as the `Superclass`. This saves time and effort, and it helps to prevent errors.
The Lombok Builder Inheritance Workaround is a valuable tool for Java developers. It can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Lombok Builder Inheritance Workaround FAQs
The following are some frequently asked questions about the Lombok Builder Inheritance Workaround:
Question 1: What is the Lombok Builder Inheritance Workaround?
The Lombok Builder Inheritance Workaround is a technique that allows subclasses to use the builder of the superclass.
Question 2: Why is the Lombok Builder Inheritance Workaround useful?
The Lombok Builder Inheritance Workaround is useful because it can save time and effort, and it can help to ensure that the code is consistent and maintainable.
Question 3: How does the Lombok Builder Inheritance Workaround work?
The Lombok Builder Inheritance Workaround works by creating a new builder class for the subclass that delegates to the builder of the superclass.
Question 4: What are the benefits of using the Lombok Builder Inheritance Workaround?
The benefits of using the Lombok Builder Inheritance Workaround include saving time and effort, improving code maintainability, increasing reusability, and enhancing extensibility.
Question 5: Are there any drawbacks to using the Lombok Builder Inheritance Workaround?
There are no major drawbacks to using the Lombok Builder Inheritance Workaround. However, it is important to note that the workaround only works with Lombok-generated builders.
Question 6: How can I learn more about the Lombok Builder Inheritance Workaround?
You can learn more about the Lombok Builder Inheritance Workaround by reading the official documentation or by searching for tutorials online.
The Lombok Builder Inheritance Workaround is a valuable tool that can save developers time and effort. It can also improve the quality of the code by reducing boilerplate code, improving code maintainability, increasing reusability, and enhancing extensibility.
Note: The Lombok Builder Inheritance Workaround is not a replacement for the Builder pattern. The Builder pattern is a design pattern that provides a flexible and extensible way to create objects. The Lombok Builder Inheritance Workaround is a technique that can be used to simplify the implementation of the Builder pattern.
Tips for Using the Lombok Builder Inheritance Workaround
The Lombok Builder Inheritance Workaround is a powerful tool that can save you time and effort. Here are a few tips for using the workaround effectively:
Tip 1: Use the workaround to avoid writing boilerplate code.
One of the main benefits of the workaround is that it can eliminate the need to write boilerplate code to create a builder class for a subclass. This can save you a significant amount of time, especially for subclasses with a large number of fields.
Tip 2: Use the workaround to improve code maintainability.
The workaround can also help you improve code maintainability by ensuring that the builder class for a subclass is consistent with the builder class for the superclass. This makes it easier to understand and maintain the code.
Tip 3: Use the workaround to increase code reusability.
The workaround can also help you increase code reusability by allowing subclasses to reuse the builder class of the superclass. This can save you time and effort when developing new code.
Tip 4: Use the workaround to enhance code extensibility.
The workaround can also help you enhance code extensibility by allowing subclasses to extend the functionality of the builder class of the superclass. This can be useful when adding new features to the code.
Tip 5: Use the workaround with caution.
It is important to note that the workaround only works with Lombok-generated builders. If you are using a different builder implementation, the workaround will not work.
Summary
The Lombok Builder Inheritance Workaround is a valuable tool that can save you time and effort. By following these tips, you can use the workaround effectively to improve the quality of your code.
Conclusion
The Lombok Builder Inheritance Workaround is a powerful technique that can save developers time and effort. The workaround allows subclasses to use the builder of the superclass, which can eliminate the need to write boilerplate code, improve code maintainability, increase code reusability, and enhance code extensibility.
The workaround is simple to implement and use. It is also compatible with the latest version of Lombok. As a result, the workaround is a valuable tool for Java developers of all skill levels.
In the future, the workaround is likely to become even more popular as more developers discover its benefits. The workaround is a valuable addition to the Lombok toolset, and it is sure to continue to be used by developers for many years to come.
Unveiling The Comedic Legacy: John Cleese's Daughter Takes The Stage
Unveiling The Truth: In-Depth Analysis Of "Is Lefty Sm Dead The Shooting Death"
Unveiling Cayden Wyatt Costner's Net Worth And Relationship: Surprising Revelations
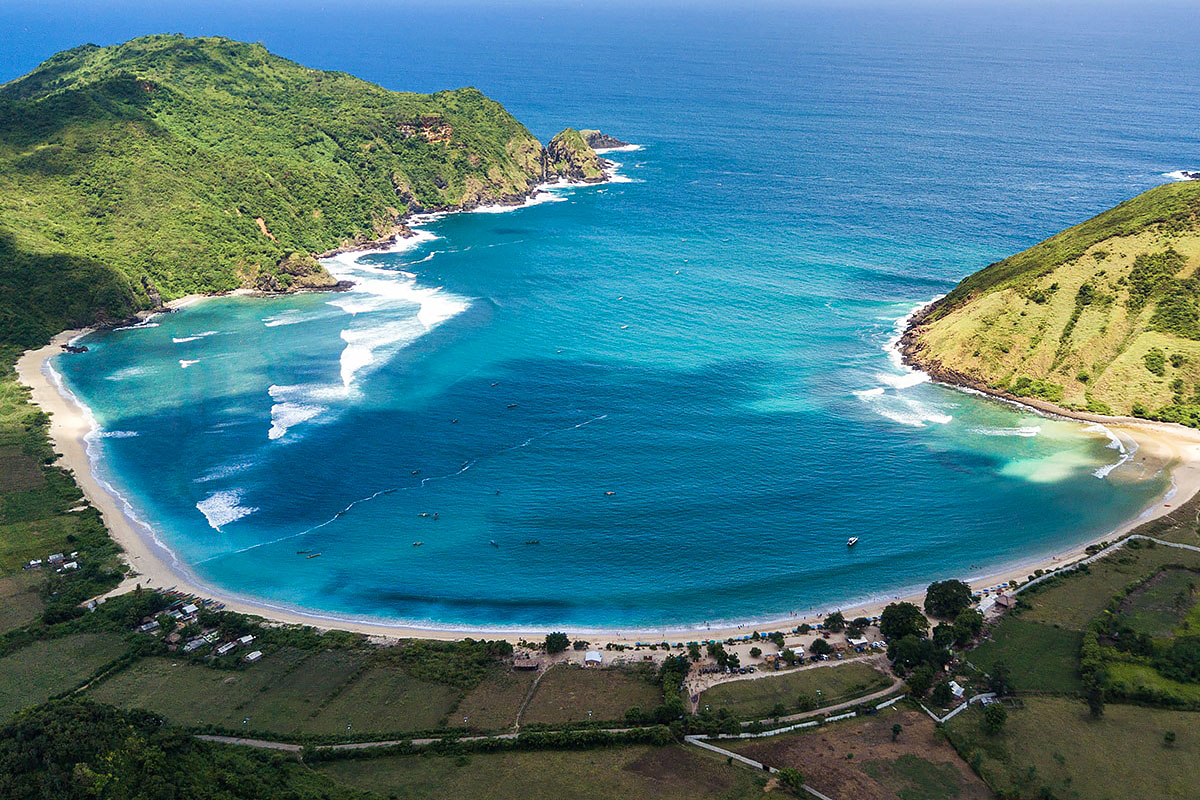
ncG1vNJzZmillaKvrbGNrGpnraNixKa%2F02ZpZ5mdlsewusCwqmebn6J8rbvMm6akZZKqtq2wxKtkoqaYmr%2BqwMCnmp5lp6S%2FrK3RqKynnF6dwa64